While there is a QuickStart example on the Streamlit site that shows how to connect to OpenAI using LangChain I thought it would make sense to create Streamlit Langchain Quickstart App with Azure OpenAI.
Please see the notes inside as the code comments
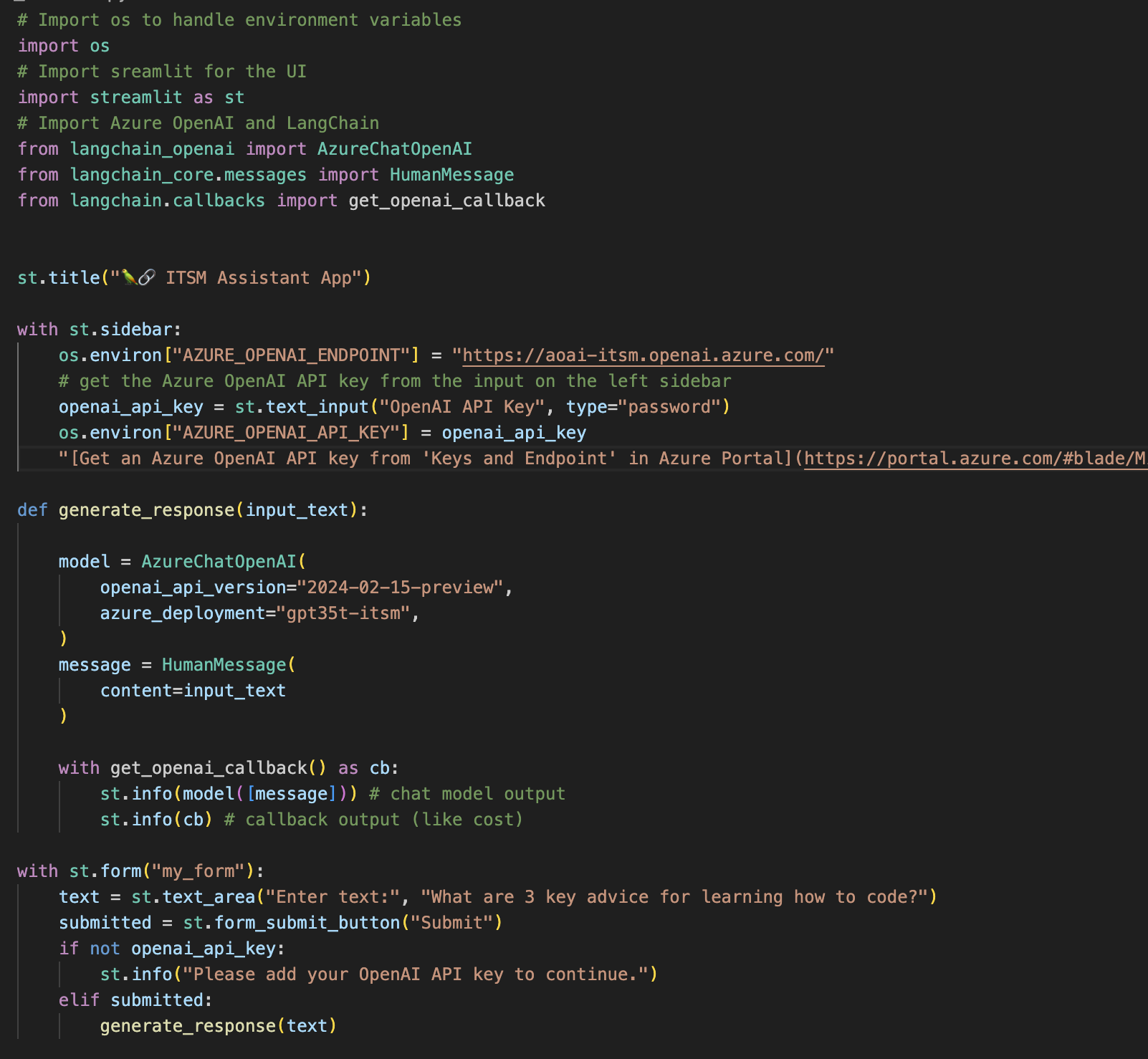
# Import os to handle environment variables import os # Import sreamlit for the UI import streamlit as st # Import Azure OpenAI and LangChain from langchain_openai import AzureChatOpenAI from langchain_core.messages import HumanMessage from langchain.callbacks import get_openai_callback st.title("🦜🔗 ITSM Assistant App") with st.sidebar: os.environ["AZURE_OPENAI_ENDPOINT"] = "https://aoai-itsm.openai.azure.com/" # get the Azure OpenAI API key from the input on the left sidebar openai_api_key = st.text_input("OpenAI API Key", type="password") os.environ["AZURE_OPENAI_API_KEY"] = openai_api_key "[Get an Azure OpenAI API key from 'Keys and Endpoint' in Azure Portal](https://portal.azure.com/#blade/Microsoft_Azure_ProjectOxford/CognitiveServicesHub/OpenAI)" def generate_response(input_text): model = AzureChatOpenAI( openai_api_version="2024-02-15-preview", azure_deployment="gpt35t-itsm", ) message = HumanMessage( content=input_text ) with get_openai_callback() as cb: st.info(model([message]).content) # chat model output st.info(cb) # callback output (like cost) with st.form("my_form"): text = st.text_area("Enter text:", "What are 3 key advice for learning how to code?") submitted = st.form_submit_button("Submit") if not openai_api_key: st.info("Please add your OpenAI API key to continue.") elif submitted: generate_response(text)
Now. a few notes:
- Model initiation will need
- AZURE_OPENAI_ENDPOINT – get if from Azure Portal > Azure OpenAI. Select your service > Keys and Endpoint
- azure_deployment – Get it from the Azure OpenAI Portal > Deployments (the value under the Deployment Name column)
- openai_api_version – the easiest way I found is to go to the Azure OpenAI Portal > Playground > Chat > View Code (in the middle top)
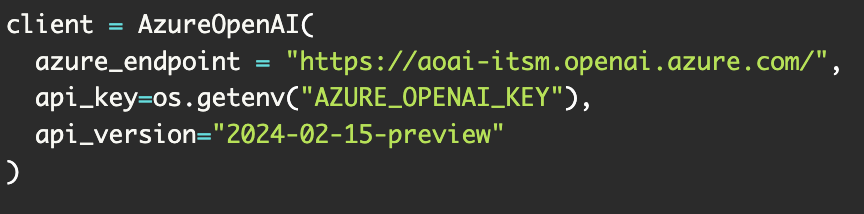